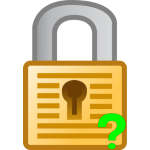
ID Ransomware
Upload a ransom note and/or sample encrypted file to identify the ransomware that has encrypted your data.
Knowing is half the battle!
API Documentation
API Authentication
All API calls to ID Ransomware require Base64-encoded HMAC-256 hashing of the request URI for authentication, and is expected to be passed as an Authorization
header in the form Key:hash
.
Here is an example call wrapper in PHP:
<?php
define('AUTH_KEY', 'your_key');
define('AUTH_SECRET', 'your_secret');
define('IDR_API', 'https://id-ransomware.malwarehunterteam.com/api');
function idr_call($path){
$url = IDR_API . $path;
$hash = hash_hmac('sha256', $url, AUTH_SECRET, true);
$base64_hash = base64_encode($hash);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($ch, CURLOPT_HEADER, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Authorization: ' . AUTH_KEY . ': ' . $base64_hash));
$response = curl_exec($ch);
if($response === false){
throw new Exception(curl_errno($ch) . ': ' . curl_error($ch));
}
curl_close($ch);
return json_decode($response);
}
?>
Please refer to this page for examples of using HMAC-256 in different languages.
-
Get Ransomwares
Returns basic data about all ransomwares supported.
URL
/ransomwares
Method
GET
URL Params
None
Success Response
-
Code:
200
Content:[{"name": "7ev3n", "status": "Not Decryptable", tag: "7EV3N"}, ...]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
Sample Call
<?php $ransomwares = idr_call('/ransomwares'); ?>
-
Code:
-
Get Ransomware Data
Returns data for a single ransomware, including status and information URL.
URL
/ransomwares/{tag}
Method
GET
URL Params
Required:
-
tag=[string]
Success Response
-
Code:
200
Content:{"name": "EnCiPhErEd", "status": "Decryptable", "info_url": "http:..."}
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
-
Code:
404
Content:{"error": "Invalid ransomware"}
Sample Call
<?php $enciphered = idr_call('/ransomwares/ENCIPHERED'); ?>
-
-
Get Extensions
Returns all extensions supported, grouped by ransomware.
URL
/ransomwares/extensions[/{regex}]
Method
GET
URL Params
Optional:
-
regex=[true|false]
Return regular expression patterns for extensions. Defaultfalse
.
Success Response
-
Code:
200
Content:[{"name": "7ev3n", "extensions": [".R5A", ".R4A"]}, ...]
-
Code:
200
Content:[{"name": "7ev3n", "extensions": ["(.*)\\.R5A", "(.*)\\.R4A"]}, ...]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
Sample Call
<?php $extensions = idr_call('/ransomwares/extensions'); ?>
-
-
Get Ransom Notes
Returns all ransom notes supported as regular expression patterns, grouped by ransomware.
URL
/ransomwares/ransomnotes
Method
GET
URL Params
none
Success Response
-
Code:
200
Content:[{"name": "Cerber", "filenames": ["# DECRYPT MY FILES #.(txt|vbs|html)"]}, ...]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
Sample Call
<?php $ransom_notes = idr_call('/ransomwares/ransomnotes'); ?>
-
Code:
-
Get Addresses
Returns all addresses supported, grouped by ransomware.
URL
/ransomwares/addresses[/{type}]
Method
GET
URL Params
Optional:
-
type=[email|bitcoin|bitmessage]
Return addresses of a specific type. Defaultfalse
.
Success Response
-
Code:
200
Content:[{"name": "Mobef", "addresses": ["[email protected]"]}, ...]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
-
Code:
404
Content:{"error": "Invalid address type"}
Sample Call
<?php $ransom_notes = idr_call('/ransomwares/addresses'); ?>
-
-
Get Extensions for Ransomware
Returns extensions for a single ransomware.
URL
/ransomwares/{tag}/extensions[/{regex}]
Method
GET
URL Params
Required:
-
tag=[string]
Optional:
-
regex=[true|false]
Return regular expression patterns for extensions. Defaultfalse
.
Success Response
-
Code:
200
Content:[".cerber"]
-
Code:
200
Content:["(.*)\\.cerber"]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
-
Code:
404
Content:{"error": "Invalid ransomware"}
Sample Call
<?php $cerber_extensions = idr_call('/ransomwares/CERBER/extensions'); ?>
-
-
Get Ransom Notes for Ransomware
Returns ransom notes for a single ransomware.
URL
/ransomwares/{tag}/ransomnotes
Method
GET
URL Params
Required:
-
tag=[string]
None
Success Response
-
Code:
200
Content:["Howto_Restore_FILES\\.(BMP|HTM|TXT)", ...]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
-
Code:
404
Content:{"error": "Invalid ransomware"}
Sample Call
<?php $teslacrypt3_ransomnotes = idr_call('/ransomwares/TESLACRYPT3/ransomnotes'); ?>
-
-
Get Addresses for Ransomware
Returns addresses for a single ransomware.
URL
/ransomwares/{tag}/addresses[/{type}]
Method
GET
URL Params
Required:
-
tag=[string]
Optional:
-
type=[email|bitcoin|bitmessage]
Return addresses of a specific type. Defaultfalse
.
Success Response
-
Code:
200
Content:[".cerber"]
-
Code:
200
Content:["[email protected]","BM-NBvzKEY8raDBKb9Gp1xZMRQpeU5svwg2"]
Error Response
-
Code:
403
Content:{"error": "Invalid API key\/secret"}
-
Code:
404
Content:{"error": "Invalid ransomware"}
-
Code:
404
Content:{"error": "Invalid address type"}
Sample Call
<?php $cerber_extensions = idr_call('/ransomwares/MOBEF/addresses'); ?>
-